Python Basics
Let’s have a quick Python 101 right now.
If you’re still thinking if Python is the way to go, check out our other articles on:
- All about Python
- What is Python?
- How people use Python?
- 4 reasons why you would want to learn Python
- Is Python for beginners?
- What to consider when learn to code?
—
You can use repl.it to run these code examples. Remember to choose Python.
Python Basics: String & Variable
Print a string (must surround with quote):
{% c-line %}print(“Learn to code at NEXT Academy”){% c-line-end %}
Output >> Learn to code at NEXT Academy
Print a variable:
{% c-block language=”js” %}
message = “Learn to code at NEXT Academy”
print(message)
{% c-block-end %}
Give it a try!
Next, change the message to something else!
Concatenate
{% c-block language=”js” %}
name = “Zoe”
message = “Learn to code at NEXT Academy”
print(“Hey ” + name + “, ” + message)
{% c-block-end %}
Give it a try!
Commonly Used Python Operators
Let’s do some math in Python.
Integer 2 + integer 3 should equal to 5 right?
{% c-line %}2 + 3{% c-line-end %}
Basic Python Arithmetic Operators
- Addition: +
- Subtraction: –
- Multiply: *
- Division: /
- Exponential: **
Give it a try: 10 + 2, 10 / 2 etc.
Use this code:
{% c-block language=”js” %}
print(10 + 2)
{% c-block-end %}
β
Python Comparison Operators
- Less than: <
- More than: >
- Equal to: ==
- Not equal to: !=
- Less than or equal to: <=
- More than or equal to: >=
Give it a try: 10 < 2
Use this code:
{% c-block language=”js” %}
print(10 < 2)
{% c-block-end %}
Python Logical Operators
- and: if both statements are true, it is true
- or: if one statements is true, it is true
- not: reverse the result. If it’s true, say false!
Use this code:
{% c-block language=”js” %}
x = 5
print(x > 3 and x < 10) # and
print(x > 3 or x < 10) # or
print(not(x > 3 or x < 10)) # not
{% c-block-end %}
Python Membership Operators
- in: if the item is in a sequence, it is true
- not in: if the item is NOT in a sequence, it is true
Use this code:
{% c-block language=”py” %}
x = [“apple”, “banana”]
print(“apple” in x)Β # in is true, because apple is in x
print(“pineapple” in x) # in is false, because there is no pineapple in x
print(“pineapple” not in x)Β # not in is true because the pineapple is not in x
{% c-block-end %}
Python List
If you want to make a list of items in a particular order. You can modify it by adding more items or taking items out.
{% c-line %}fruits = [“apple”, “pear”, “banana”]{% c-line-end %}
Use this code:
{% c-block language=”js” %}
fruits = [“apple”, “pear”, “banana”]
fruits.append(“durian”)
print(fruits)
{% c-block-end %}
Python Tuple
It is similar to a list but you can’t modify it.
{% c-line %}primaryColors = (“red”, “yellow”, “blue”){% c-line-end %}
Python Dictionary
It stores connections between pieces of information. It comes in a pair, which is a key, and a value.
{% c-line %}zoe = { ‘gender’: ‘f’, ‘age’: ‘prefer not to say’}{% c-line-end %}
Use this code:
{% c-block language=”js” %}
zoe = { ‘gender’: ‘f’, ‘age’: ‘prefer not to say’}
print(“What is Zoe’s age? ” + zoe[‘age’])
{% c-block-end %}
What’s next?
Now that you’ve learned different Python data types, there are actually more of them which can be used for different scenarios which would make sense to you when you start coding.
After data types, there are other things to look into, such as:
- Conditional statements
- Loops
Let’s quickly run through a simple exercise:
In a human mind: Bring an umbrella when it is raining and a hat when it is hot.
You need to give the computer instructions in this logical systematic way:
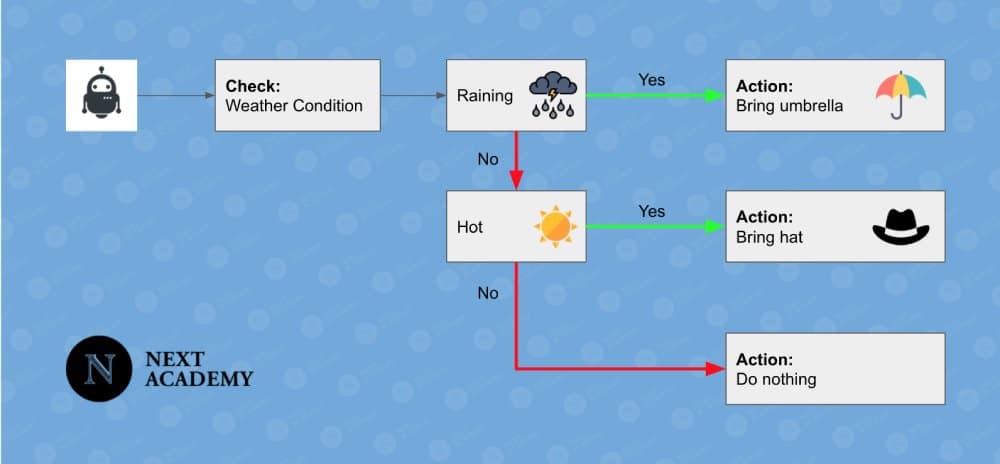
Use this code:
{% c-block language=”js” %}
weather = “hot”
# The logical statement for the output
if weather == “raining”:
Β Β Β print(“Bring umbrella”)
elif weather == “hot”:
Β Β Β Β print(“Bring hat”)
else:
Β Β Β Β print(“Do nothing”)
{% c-block-end %}
β
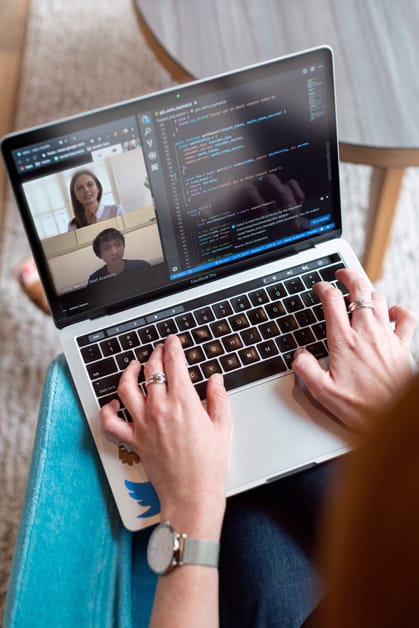
β
See how you have to implement code in a step-by-step manner?
I have this is a good quick teaser on what you can do with code.
If you want to take it to the next level, why not try out how to write an algo trading bot to automate your investment, or if you are serious about learning how to code and build apps, join our 10-week Full Stack Web Development Bootcamp.
-
Josh Tenghttps://www.nextacademy.com/author/josh/
-
Josh Tenghttps://www.nextacademy.com/author/josh/
-
Josh Tenghttps://www.nextacademy.com/author/josh/
-
Josh Tenghttps://www.nextacademy.com/author/josh/